Convert Date to String: A Comprehensive Guide
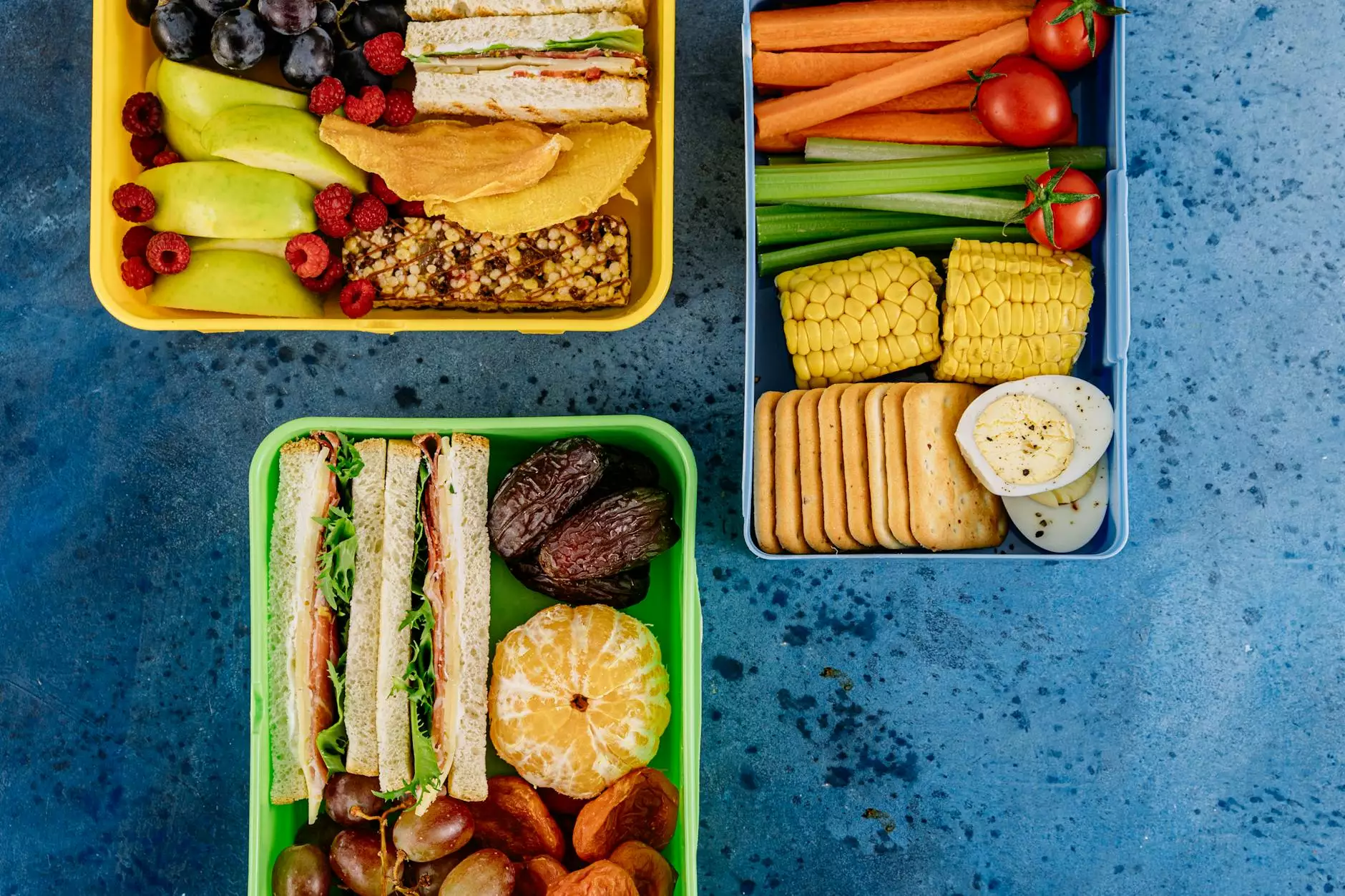
In the world of programming and data manipulation, the ability to convert date to string is a crucial skill. This process is highly valuable in various business applications, including web design and software development. Understanding how to effectively convert dates to strings can enhance data presentation, improve user interfaces, and facilitate better data processing in your projects. In this article, we will explore various programming languages, delve into practical implementations, and highlight the significance of this conversion in business settings.
Understanding the Importance of Converting Dates to Strings
Data, especially dates, often needs to be displayed in a format that is human-readable and contextually relevant. Converting dates to strings allows developers to:
- Present Data Clearly: Display dates in formats that users can easily read and understand, such as "March 1, 2023" instead of "2023-03-01."
- Customize Date Formats: Tailor date displays to suit diverse regional expectations, accounting for cultural differences in date presentation.
- Enhance Data Storage: Store dates in a consistent string format in databases, ensuring uniformity and ease of access.
- Improve User Experience: Provide a more intuitive interface for applications that rely heavily on date manipulations.
How to Convert Date to String in Various Programming Languages
The method of converting dates to strings varies across different programming languages. Below is a detailed overview of how to achieve this in popular languages like Python, JavaScript, Java, and C#.
Converting Dates to Strings in Python
Python is renowned for its simplicity and readability, making it a popular choice for developers. Here’s how you can convert a date to a string in Python:
from datetime import datetime # Convert date to string date = datetime.now() date_string = date.strftime("%Y-%m-%d")In this snippet:
- We import the datetime module.
- The strftime method formats the date to a string representation in "YYYY-MM-DD" format.
Converting Dates to Strings in JavaScript
JavaScript plays a crucial role in web design, especially for front-end development. Here is how to convert a date to a string using JavaScript:
// Convert date to string let date = new Date(); let dateString = date.toISOString(); // or use date.toDateString() for a different formatIn this code snippet:
- A new date object is created.
- The toISOString method returns a string in the simplified extended ISO format (YYYY-MM-DDTHH:mm:ss.sssZ).
Converting Dates to Strings in Java
Java, a language favored for its portability, provides robust date manipulation capabilities. Here’s how you can convert dates to strings in Java:
import java.text.SimpleDateFormat; import java.util.Date; // Convert date to string Date date = new Date(); SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd"); String dateString = formatter.format(date);In this example:
- We import the necessary classes from the java.text and java.util packages.
- SimpleDateFormat is used to format the date in a readable string format.
Converting Dates to Strings in C#
C# is a versatile language used extensively in enterprise applications. Here’s how to convert a date to a string in C#:
using System; // Convert date to string DateTime date = DateTime.Now; string dateString = date.ToString("yyyy-MM-dd");This snippet illustrates:
- Creating a DateTime instance representing the current date and time.
- Using the ToString method with a format string to obtain the date in "YYYY-MM-DD" format.
Why Businesses Need to Convert Dates to Strings
Every business relies on data to drive decisions, create reports, and interact with customers. Here are some reasons why converting dates to strings is particularly beneficial for businesses:
- Data Consistency: Ensuring that dates are consistently formatted across systems prevents data misinterpretation and enhances data integrity.
- Effective Reporting: Reporting tools often require dates in string format for ease of display and analysis, allowing for more effective data storytelling.
- Integration Across Platforms: Many businesses operate on multiple platforms or languages. Converting dates to strings allows for smoother data transfers and integrations between systems.
- Improved Customer Interaction: Applications and services can display relevant dates to customers in formats they understand, improving user engagement and satisfaction.
Best Practices for Converting Dates to Strings
To ensure accurate and effective date conversions, consider the following best practices:
- Choose the Right Format: Understand regional date formats, such as "DD/MM/YYYY" vs "MM/DD/YYYY", and choose the appropriate format for your audience.
- Validate User Inputs: Implement checks to ensure that any user-entered dates are valid before conversion to prevent errors in your application.
- Utilize Libraries: In many programming languages, libraries exist that can simplify and standardize date conversions.
- Be Aware of Time Zones: Ensure that string representations of dates account for time zones to avoid confusion, especially in global applications.
Conclusion
Mastering the ability to convert date to string is an essential skill for developers working in web design and software development. It's not only about making data readable; it's about creating a seamless experience for users and maintaining the integrity of business data.
By understanding how to convert dates across different programming languages and adhering to best practices, businesses can enhance their applications and foster better user experiences. Embracing these skills will ultimately lead to more effective data management and improved customer satisfaction.
At semalt.tools, we specialize in delivering innovative solutions in web design and software development, integrating best practices in data manipulation to empower your business.